Slider Card
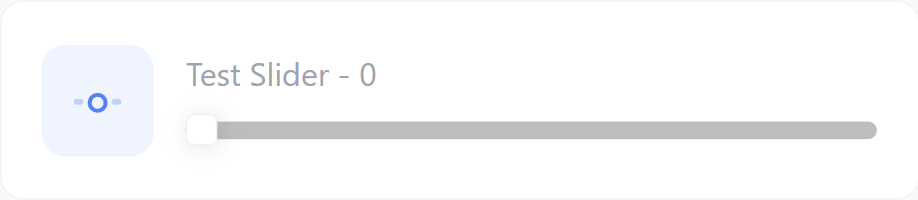
An interactive horizontal slider component for selecting numeric values within a defined range. Ideal for controlling incremental values like brightness levels or other adjustable numeric parameters on the dashboard.
Initializer
With slider card, Initializer requires min
& max
range. Additionally you can also supply step
as a seventh argument to set the step size of the slider.
/*
Slider Card
Valid Arguments: (ESPDash dashboard, Card Type, const char* name, const char* symbol (optional), int min, int max)
*/
Card card1(&dashboard, SLIDER_CARD, "Test Slider", "", 0, 255, 1);
Callback
Slider Card requires a callback function which will be called when we receive a input from our dashboard. We will be calling our attachCallback
function and provide a lambda function with a int
argument.
You need to call the update
function and sendUpdates
immediately once you receive the value in callback. Otherwise user input will not be registered on dashboard.
/*
We provide our attachCallback with a lambda function to handle incomming data
`value` is the boolean sent from your dashboard
*/
card1.attachCallback([&](float value){
Serial.println("[Card1] Slider Callback Triggered: "+String(value));
card1.update(value);
dashboard.sendUpdates();
});
Updater
card1.update(int value);
card1.update(float value);
Or, update symbol along your value:
card1.update(244, "%");
Reference
This is a reference sketch showing positions for intializer, callback and updater.
...
/* Slider card initializer */
Card slider(&dashboard, SLIDER_CARD, "Test Slider", "", 0, 255, 1);
void setup() {
...
/* Slider card callback */
slider.attachCallback([&](float value){
Serial.println("Slider Callback Triggered: "+String(value));
/* Slider card updater - you need to update the slider with latest value upon firing of callback */
slider.update(value);
/* Send update to dashboard */
dashboard.sendUpdates();
});
}
void loop() {
...
}